Hello, this is my first post on my blog. I have been thinking about creating a blog for a year, but a lack of time didn’t allow me to do so.
So, you are here because you are looking for a way to train yourself to beat the code challenges that prevent you from getting a good job, aren’t you?
I have seen so many people on Reddit asking for help on how to solve code challenges. Some of the posts say something like this: “I have solved many code challenges on [your favorite practice platform], but I’m still not getting my dream job.”
It doesn’t matter if you solve a lot of them or practice every day if YOU DON’T UNDERSTAND THE BASICS OR TECHNIQUES to solve those challenges.
I have been interviewing for a few weeks (and months), and I have noticed something… let me tell you that most of the code challenges are of medium level and are related to arrays (sorting or manipulation). After practicing, I have noticed that there are common techniques I have discovered to solve many of the problems that companies use in their technical interviews (except if you are applying to a FAANG company), and sadly no tutorial talks about them (many books describe the problems and provide the code solution without explaining it line by line).
Let’s dive in deep on this:
Storing Variable
Problems like “merge overlapping intervals” (a common problem for technical interviews) require not only understanding when intervals are overlapping but also starting with storing a variable that will contain the last element used to compare with the next one. This pattern could help you in this and many other problems related to arrays.
ranges = [[1,2], [3,4],[5,10]]
help_variable = None
for elem in ranges:
if help_variable is None:
help_variable = elem
continue
In this case, help_variable
is the storing variable. As you can see, we save the first element. In the next iteration, we can do whatever we want with [1, 2] and [3, 4]. In the case of the merging overlapping intervals problem, we need to compare the last element of help_variable
with the first of the current one (help_variable[1] > elem[0]
) in order to know if there’s an overlap.
Adding one to Index
Some problems need to compare the current value with the next one. If you are a beginner manipulating arrays, perhaps you are having problems with this, especially when you have someone watching your screen or code and judging you.
The main key is getting the total array elements and subtracting 1 from the total so you can get the next element in your array. (I know Python lists are not arrays, but let’s use the word “array.”) array[i] > array[i + 1]
.
my_list = [1,2,3,4,5]
for i in range(len(my_list) -1):
if my_list[i] < my_list[i + 1]:
print("The next element is greather than current one")
As you can see, I have highlighted range
and len
. After getting the length of my_list
, I subtracted one from it. This way, I could see the next value in the list/array by adding one to the index (i)
.
Basic, right? but powerful
Adding two to the Index
What about knowing the two elements right next to the current one? If you need to access the two elements to the right of the current one, you need to use the following pattern:
my_list = [1,2,3,4,5,5,9]
for i in range(len(my_list)):
if (i+2 < len(my_list)) and (my_list[i] < my_list[i + 2]):
print("i + 2 = ",my_list[i+2]," is greater than current one = ", my_list[i])
This will print:
i + 2 = 3 is greater than the current one = 1
i + 2 = 4 is greater than the current one = 2
i + 2 = 5 is greater than the current one = 3
i + 2 = 5 is greater than the current one = 4
i + 2 = 9 is greater than the current one = 5
As you can see, we are comparing the current element with the one located two places to the right. It has been possible thanks to the highlighted line. We need to check that i + 2
is less than the list/array length so we can compare the current element with the one located at i + 2
.
Easy peasy!!
Finally…
Traversing Matrices
In college, you probably learned how to traverse a matrix. If you don’t remember, you are about to be reminded.
Let’s write a new 3×3 matrix to use in our examples:
my_matrix = [
[1,2,3]
[2,3,4]
[3,4,5]
]
Knowing the number of rows in the matrix
Remember, you need to get the length of the matrix:
rows = len(my_matrix)
Knowing the number of cols in the matrix
To obtain the number of cols you are going to get the length of the first element in the matrix:
cols = len(my_matrix[0])
Getting the number of cols and rows could be important for your problem-solving algorithm. Keep these examples in mind.
Traversing Rows
Most of the time we are going to traverse rows and i think is the most accepted or used way when trying to solve matrix problems:
for row in range(rows):
for col in range(cols):
print(“traversing rows=”, my_matrix[row][col])
Traversing Cols.
if you want to traverse the cols and handle elements vertically (as vectors) you only have to change the order of the fors loops:
for col in range(cols):
for row in range(rows):
print(“traversing cols=”, my_matrix[row][col])
The End
Well, this is really basic stuff but if you are new with code challenges with arrays this will really be helpful for you.
Last week, I had the “merge overlapping interval” problem but didn’t study at all, so… I failed miserably. It was my main motivation to “study” and write this post. 🙂
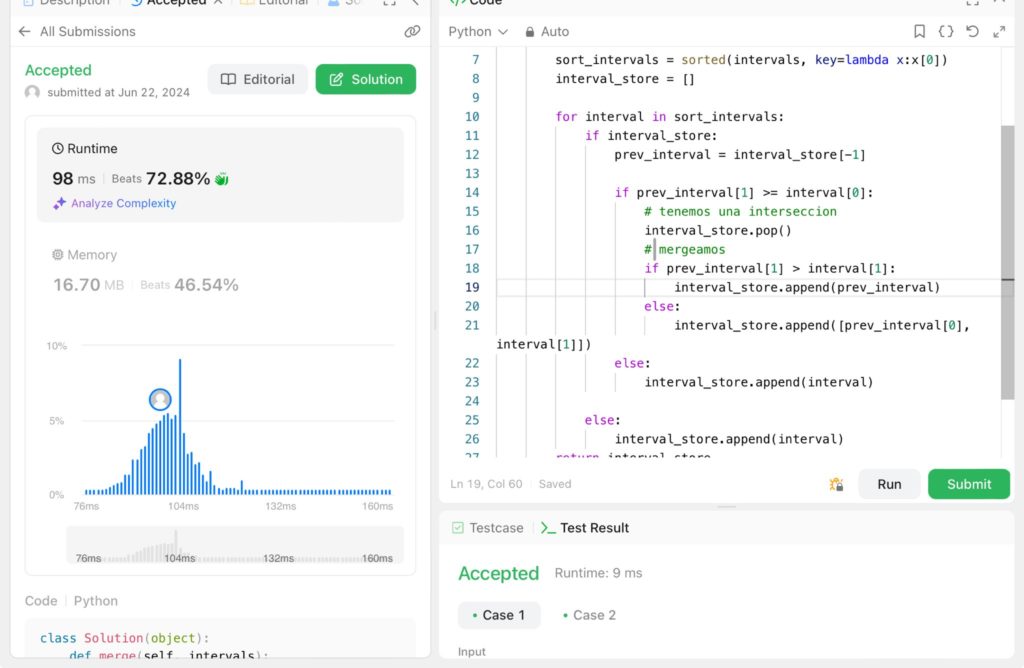
(Reduced the runtime a bit more but didn’t take screenshot)
Comments